Python Global Variables
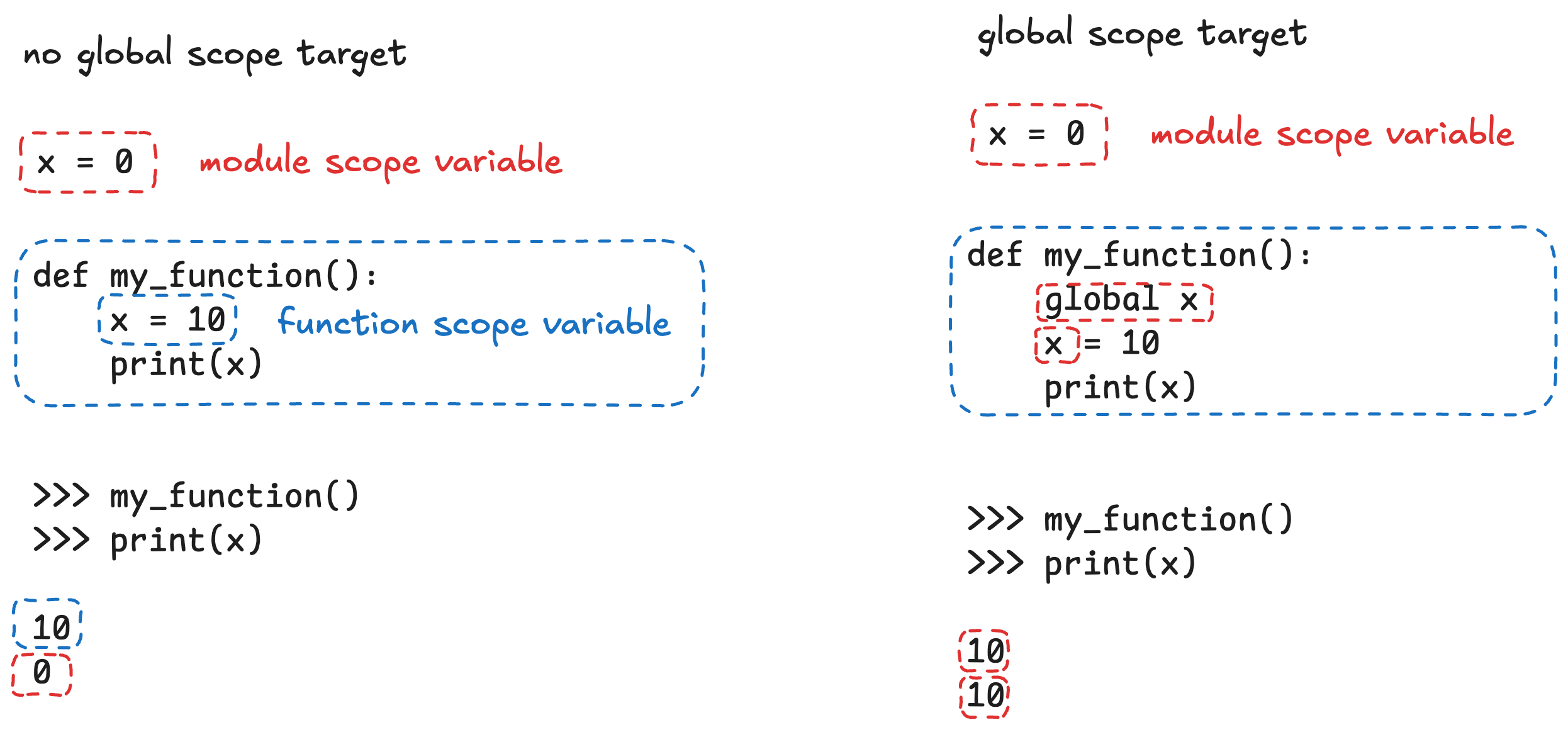
global keyword
In Python, a global variable is a variable that is defined outside of any function or class and is available for use throughout the entire program. Global variables are defined using the global keyword, which specifies that a particular variable is a global variable rather than a local variable.
Here is an example of how to define and use a global variable in Python:
x = 10 # x is a global variable def some_function(): print(x) # we can access and use the global variable x inside the function some_function() # prints 10
It's important to note that global variables can be accessed and modified from within any function or class in the program. However, if you want to modify a global variable inside a function, you must use the global keyword to specify that you are modifying the global variable, as shown in the following example:
x = 10 # x is a global variable def some_function(): global x # specify that we are modifying the global variable x x = 20 # modify the value of x some_function() print(x) # prints 20
It's generally considered good programming practice to use global variables sparingly, as they can make it difficult to understand and debug your code. In most cases, it's better to pass values as arguments to functions or to define variables within functions as local variables, unless you have a specific reason to use a global variable.
Examples
Example 1: Accessing a global variable from within a function:
x = 10 # x is a global variable def some_function(): print(x) # we can access and use the global variable x inside the function some_function() # prints 10
Example 2: Modifying a global variable from within a function:
x = 10 # x is a global variable def some_function(): global x # specify that we are modifying the global variable x x = 20 # modify the value of x some_function() print(x) # prints 20
Example 3: Accessing a global variable from within a class method:
x = 10 # x is a global variable class SomeClass: def some_method(self): print(x) # we can access and use the global variable x inside the class method obj = SomeClass() obj.some_method() # prints 10
Example 4: Modifying a global variable from within a class method:
x = 10 # x is a global variable class SomeClass: def some_method(self): global x # specify that we are modifying the global variable x x = 20 # modify the value of x obj = SomeClass() obj.some_method() print(x) # prints 20
Best Practices
- Avoid using global variables whenever possible: Global variables can make your code difficult to understand and maintain, because they can be accessed and modified from anywhere in your code. Instead, try to use local variables and pass values between functions as needed.
- Use global variables sparingly: If you do need to use global variables, use them sparingly and only when necessary. Overuse of global variables can lead to confusing code and make it difficult to track changes.
- Declare global variables at the top of your code: To make it clear that a variable is a global variable, it's a good idea to declare it at the top of your code, outside of any functions or classes. This makes it easy to see which variables are global and which are local.
- Use descriptive names for global variables: It's important to give your global variables descriptive names so that it's clear what they are used for. This will make it easier for others (and yourself) to understand your code.
- Protect global variables from modification: If you don't want a global variable to be modified, you can use the global keyword to declare it as a read-only global variable. This will prevent accidental modifications to the variable, which can cause bugs and other issues in your code.
- Use caution when modifying global variables: When modifying global variables, be sure to understand the potential impacts on other parts of your code. Modifying a global variable can have unintended consequences, so make sure you understand how your changes will affect the rest of your code before making any modifications.