How do I count the occurrences of a list item
TL;DR
Use count()
if you search the occurrences count for a single item. Use Counter
or your own implementation if you search the count for multiple items
Context
Python has the built-in count()
method which returns the number of items with the specified value.
>>> my_list = [1,2,3,4,5,1,2,5] >>> print(my_list.count(5)) 2
The method runs in linear time (n). You should therefore try to avoid usages like the code below
>>> my_list = [1,2,3,4,5,1,2,5] >>> print(my_list.count(1)) >>> print(my_list.count(2)) >>> print(my_list.count(3)) >>> print(my_list.count(4)) >>> print(my_list.count(5))
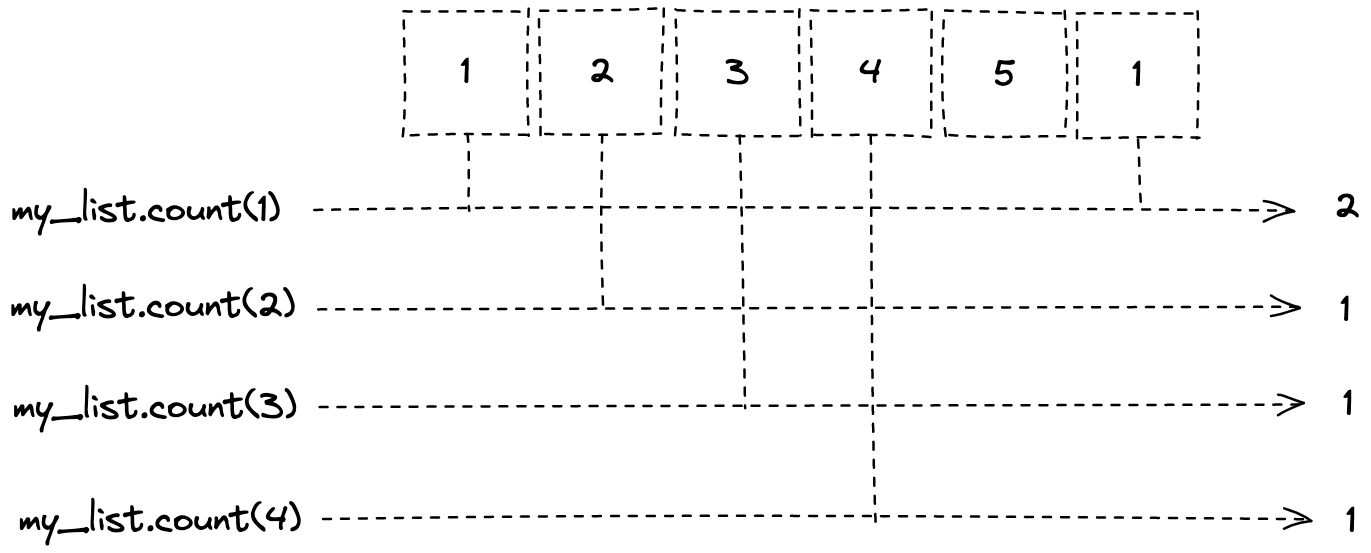
The linear runtime nature of count()
means that all values of the list are checked with each method call. This is fine if you are looking for the count of a single value but the runtime becomes quadratic if you are searching for the count for all the items in your list.
You can also find the count of all items in linear runtime.
# Find the occurrences count of 1,2,3,4,5 my_list = [1,2,3,4,5,1,2,5] count_values = { 1: 0, 2: 0, 3: 0, 4: 0, 5: 0 } for value in my_list: if value in count_values: count_values[value] += 1 >>> print(count_values[1]) >>> print(count_values[2]) 2 2