What is the difference between append and extend
Remember Me
- use
append()
to add one single item at the end of your list - use
extend()
to append the items of the specified iterable list to your list
Context
my_list.append(my_object)
adds my_object
at the end of my_list
. my_list.extend(another_list)
extends my_list
by adding the items of another_list
to my_list
.
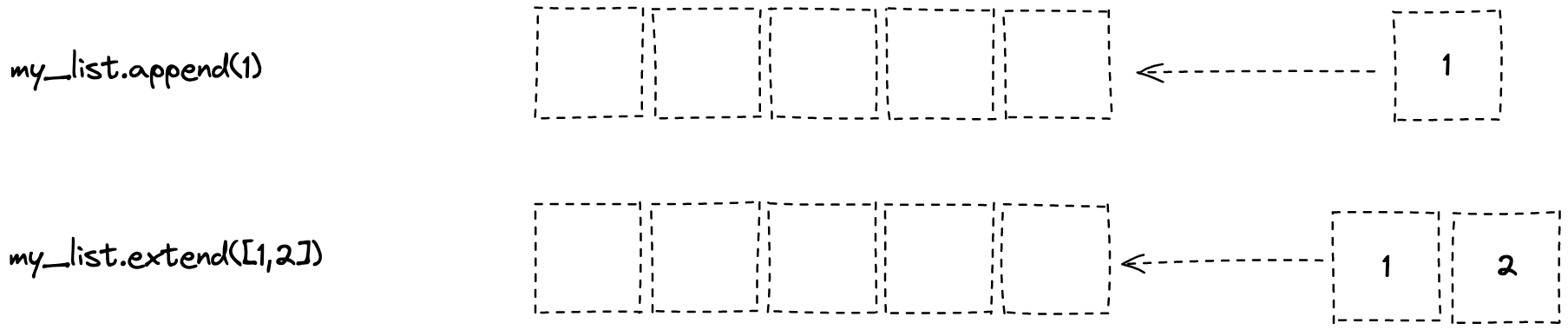
A common mistake is accidentally using append
instead of extend
which is still valid Python code
# append another list my_list = [1,2,3] my_list.append([4,5]) print(my_list) [1,2,3, [4,5]]
Use Case Examples
# filter out values larger than the threshold value def filter_out(values: List[int], threshold_value: int) -> List[int]: filtered_out_values = [] for value in values: if value >= threshold_value: filtered_out_values.append(value) return filtered_out_values >>> print(filter_out([50,20,40,10,60,55], 30)) [50,40,60,55]
"""Collect all car brands from the different countries. Assume that the followings are defined countries: list of country names request_brands: function to get the brands of the specific country filter_brands_out: function to filter out brands based on category """ car_brands = [] for country in countries: brands_from_country = request_brands(country) country_car_brands = filter_brands_out(brands_from_country, filter_category="car") car_brands.extend(country_car_brands)