How to Check if a Value Exists in a List in Python (Visualized)
In Python, there are several ways to check if a value exists in a list. Here are some common methods:
Method 1: Using the in operator
The in operator is a simple and concise way to check if a value exists in a list. It returns True if the value is in the list, and False if it is not.
Here is an example:
numbers = [1, 2, 3, 4, 5] # Check if 3 is in the list if 3 in numbers: print("3 is in the list") else: print("3 is not in the list")
3 is in the list
Note: Every time you use the in
operator, Python will iterate through the list from start to potentially the end. You should convert your list to a set if you need to check for membership multiple times: lookup = set(my_list)
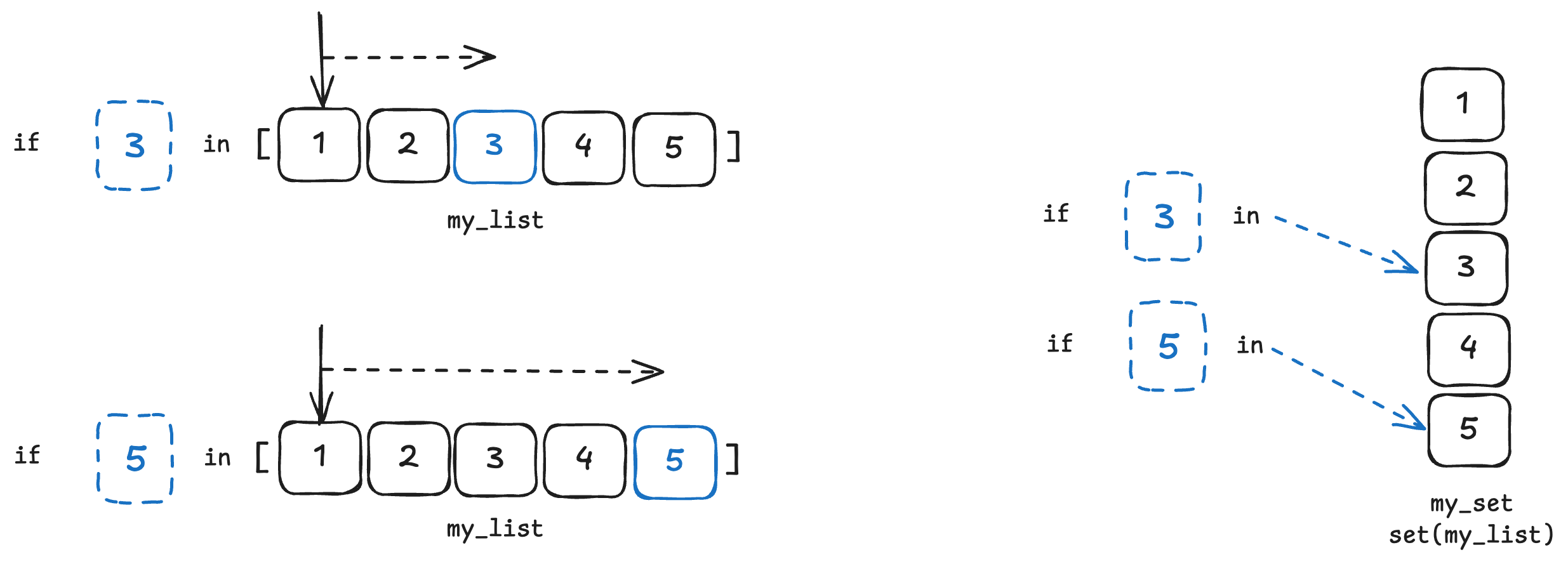
Method 2: Using the index() method
The index() method returns the index of the first occurrence of a value in a list. If the value is not in the list, it will raise a ValueError exception.
We can use this method to check if a value exists in a list by using a try-except block to handle the ValueError exception.
Here is an example:
numbers = [1, 2, 3, 4, 5] # Check if 3 is in the list try: index = numbers.index(3) print("3 is in the list at index", index) except ValueError: print("3 is not in the list")
3 is in the list at index 2
Method 3: Using the count() method
The count() method returns the number of occurrences of a value in a list. If the value is not in the list, it will return 0.
We can use this method to check if a value exists in a list by checking if the count is greater than 0.
Here is an example:
numbers = [1, 2, 3, 4, 5] # Check if 3 is in the list if numbers.count(3) > 0: print("3 is in the list") else: print("3 is not in the list")
3 is in the list
Method 4: Using a for loop
We can also use a for loop to iterate through the list and check if the value exists. This method is not as efficient as the others, but it is a good option if you need to perform additional processing on the list elements.
Here is an example:
numbers = [1, 2, 3, 4, 5] # Check if 3 is in the list found = False for number in numbers: if number == 3: found = True break if found: print("3 is in the list") else: print("3 is not in the list")
3 is in the list